how to use regular expression to match strings followed by some keyword and multiple lines
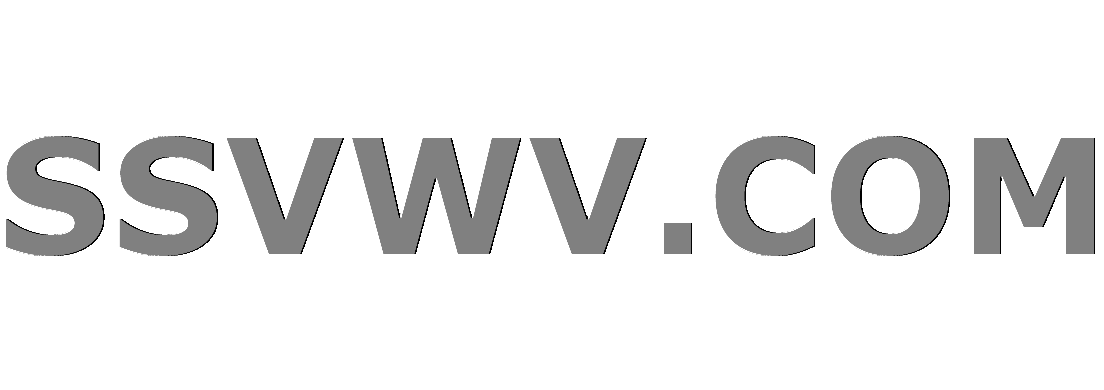
Multi tool use
up vote
-2
down vote
favorite
I have a string like this:
...
xxxx
xxx
keyword1 xxxxx
xxxx
xxxx
xxxxx keyword2 yyyy
xxx
xxxx
xxx
...
where the x and y's are just random chars. I need to match the first "keyword2 yyyy" that appears after keyword1, and there may be multiple lines after keyword1. How do I write the regular expression? Thanks!
regex string pattern-matching matching
add a comment |
up vote
-2
down vote
favorite
I have a string like this:
...
xxxx
xxx
keyword1 xxxxx
xxxx
xxxx
xxxxx keyword2 yyyy
xxx
xxxx
xxx
...
where the x and y's are just random chars. I need to match the first "keyword2 yyyy" that appears after keyword1, and there may be multiple lines after keyword1. How do I write the regular expression? Thanks!
regex string pattern-matching matching
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I have a string like this:
...
xxxx
xxx
keyword1 xxxxx
xxxx
xxxx
xxxxx keyword2 yyyy
xxx
xxxx
xxx
...
where the x and y's are just random chars. I need to match the first "keyword2 yyyy" that appears after keyword1, and there may be multiple lines after keyword1. How do I write the regular expression? Thanks!
regex string pattern-matching matching
I have a string like this:
...
xxxx
xxx
keyword1 xxxxx
xxxx
xxxx
xxxxx keyword2 yyyy
xxx
xxxx
xxx
...
where the x and y's are just random chars. I need to match the first "keyword2 yyyy" that appears after keyword1, and there may be multiple lines after keyword1. How do I write the regular expression? Thanks!
regex string pattern-matching matching
regex string pattern-matching matching
edited Nov 7 at 6:39
asked Nov 7 at 6:22
user2577547
185
185
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
-1
down vote
accepted
You can use this regex,
^(?s).*keyword1.*?(keyword2 yyyy).*$
Explanation:
- ^ --> start of string
- (?s) --> Enables dot to match new lines
- .* keyword1.*? --> Matches a string that contains keyword1 preceded and succeeded by any characters doing non-greedy match
- (keyword2 yyyy) --> matches the string of your interest
- .*$ --> followed by any characters and finally end of input
Demo
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
|
show 1 more comment
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
accepted
You can use this regex,
^(?s).*keyword1.*?(keyword2 yyyy).*$
Explanation:
- ^ --> start of string
- (?s) --> Enables dot to match new lines
- .* keyword1.*? --> Matches a string that contains keyword1 preceded and succeeded by any characters doing non-greedy match
- (keyword2 yyyy) --> matches the string of your interest
- .*$ --> followed by any characters and finally end of input
Demo
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
|
show 1 more comment
up vote
-1
down vote
accepted
You can use this regex,
^(?s).*keyword1.*?(keyword2 yyyy).*$
Explanation:
- ^ --> start of string
- (?s) --> Enables dot to match new lines
- .* keyword1.*? --> Matches a string that contains keyword1 preceded and succeeded by any characters doing non-greedy match
- (keyword2 yyyy) --> matches the string of your interest
- .*$ --> followed by any characters and finally end of input
Demo
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
|
show 1 more comment
up vote
-1
down vote
accepted
up vote
-1
down vote
accepted
You can use this regex,
^(?s).*keyword1.*?(keyword2 yyyy).*$
Explanation:
- ^ --> start of string
- (?s) --> Enables dot to match new lines
- .* keyword1.*? --> Matches a string that contains keyword1 preceded and succeeded by any characters doing non-greedy match
- (keyword2 yyyy) --> matches the string of your interest
- .*$ --> followed by any characters and finally end of input
Demo
You can use this regex,
^(?s).*keyword1.*?(keyword2 yyyy).*$
Explanation:
- ^ --> start of string
- (?s) --> Enables dot to match new lines
- .* keyword1.*? --> Matches a string that contains keyword1 preceded and succeeded by any characters doing non-greedy match
- (keyword2 yyyy) --> matches the string of your interest
- .*$ --> followed by any characters and finally end of input
Demo
answered Nov 7 at 6:25
Pushpesh Kumar Rajwanshi
2,0311716
2,0311716
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
|
show 1 more comment
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Thank you Pushpesh! I made a mistake in my original post. There are lines before and after the interested parts but I'm only interested in the "keyword2 yyyy" part. Now I have 'keyword1(?s).*?(keyword2.*?)n', but this returns the whole string starts from keyword1: keyword1 xxxxx xxxx xxxx xxxxx keyword2 yyyy How do I just extract the "keyword2 yyyy"?
– user2577547
Nov 7 at 6:46
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
Hey, you can't move (?s) part in middle of regex as that is a flag in regex which tells dot can match new lines. I think you are capturing whole regex match. For capturing the interested text, you need to capture group 1's content.
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:00
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
If I don't move the (?s) in the middle it won't match anything because there 're multiple likes between keyword1 and keyword2. How do I get capture group 1's content?
– user2577547
Nov 7 at 7:34
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
(?s) is a global flag enabler. I think you are confusing it little bit with s. For capturing group 1's content your language must be providing some way. Like in Java, you write matcherObject.group(1). What language are you dealing with?
– Pushpesh Kumar Rajwanshi
Nov 7 at 7:39
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
I'm using ANSI SQL. If I don't use (?s) it won't match the multiple lines, right?
– user2577547
Nov 7 at 21:35
|
show 1 more comment
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53184476%2fhow-to-use-regular-expression-to-match-strings-followed-by-some-keyword-and-mult%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
P,DpZId6P,tokzYW nks6qS3KqU,9rYHvd