Value from async function in URL inside Javascript fetch
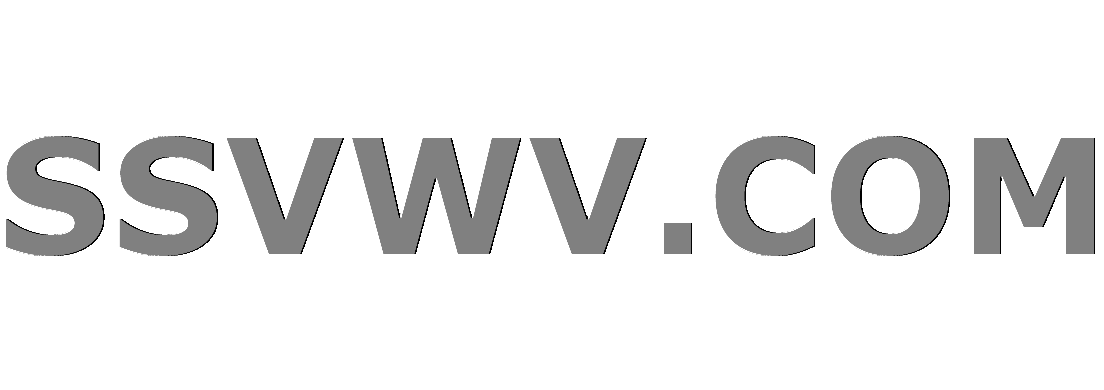
Multi tool use
up vote
1
down vote
favorite
The below code is inside a react native application:
Let's say I have the below function in API.js file:
export function getUsers() {
return fetch(BASE_URL+'/users')
.then(response => {
return response;
})
}
My application's BASE URL is dynamic or can be customized by the user. Which means, I am keeping this value in AsyncStorage of my application.
Which means, AsyncStorage.getItem('BASE_URL') holds the value for my actual BASE_URL. Problem is, the getItem function itself is a promise function. That is only wrapping it to a promise syntax will make it work. For example,
AsyncStorage.getItem('BASE_URL').then(url => {
console.log(url) //gets the URL here properly
});
My problem is, how to actually replace the BASE_URL variable in the fetch request with this value?
Remember, API.js file is not a 'component'. It just holds export functions for different API endpoints.
javascript reactjs react-native
add a comment |
up vote
1
down vote
favorite
The below code is inside a react native application:
Let's say I have the below function in API.js file:
export function getUsers() {
return fetch(BASE_URL+'/users')
.then(response => {
return response;
})
}
My application's BASE URL is dynamic or can be customized by the user. Which means, I am keeping this value in AsyncStorage of my application.
Which means, AsyncStorage.getItem('BASE_URL') holds the value for my actual BASE_URL. Problem is, the getItem function itself is a promise function. That is only wrapping it to a promise syntax will make it work. For example,
AsyncStorage.getItem('BASE_URL').then(url => {
console.log(url) //gets the URL here properly
});
My problem is, how to actually replace the BASE_URL variable in the fetch request with this value?
Remember, API.js file is not a 'component'. It just holds export functions for different API endpoints.
javascript reactjs react-native
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
The below code is inside a react native application:
Let's say I have the below function in API.js file:
export function getUsers() {
return fetch(BASE_URL+'/users')
.then(response => {
return response;
})
}
My application's BASE URL is dynamic or can be customized by the user. Which means, I am keeping this value in AsyncStorage of my application.
Which means, AsyncStorage.getItem('BASE_URL') holds the value for my actual BASE_URL. Problem is, the getItem function itself is a promise function. That is only wrapping it to a promise syntax will make it work. For example,
AsyncStorage.getItem('BASE_URL').then(url => {
console.log(url) //gets the URL here properly
});
My problem is, how to actually replace the BASE_URL variable in the fetch request with this value?
Remember, API.js file is not a 'component'. It just holds export functions for different API endpoints.
javascript reactjs react-native
The below code is inside a react native application:
Let's say I have the below function in API.js file:
export function getUsers() {
return fetch(BASE_URL+'/users')
.then(response => {
return response;
})
}
My application's BASE URL is dynamic or can be customized by the user. Which means, I am keeping this value in AsyncStorage of my application.
Which means, AsyncStorage.getItem('BASE_URL') holds the value for my actual BASE_URL. Problem is, the getItem function itself is a promise function. That is only wrapping it to a promise syntax will make it work. For example,
AsyncStorage.getItem('BASE_URL').then(url => {
console.log(url) //gets the URL here properly
});
My problem is, how to actually replace the BASE_URL variable in the fetch request with this value?
Remember, API.js file is not a 'component'. It just holds export functions for different API endpoints.
javascript reactjs react-native
javascript reactjs react-native
asked 2 days ago
Jithesh Kt
4443725
4443725
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago
add a comment |
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You can use promise chaining, where the result of AsyncStore#getItem
will be used as input to fetch
call. The linked document explain the situation that you are facing quite nicely
A common need is to execute two or more asynchronous operations back to back, where each subsequent operation starts when the previous operation succeeds, with the result from the previous step. We accomplish this by creating a promise chain.
So, changes that you need in you given code is
export function getUsers() {
return AsyncStorage.getItem('BASE_URL').then(url => {
return fetch(url+'/users').then(response => response.json());
});
}
You can use the getUsers()
without worrying about the BASE_URL
. This also ensures the separation of concern.
Hope this will help!
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can use promise chaining, where the result of AsyncStore#getItem
will be used as input to fetch
call. The linked document explain the situation that you are facing quite nicely
A common need is to execute two or more asynchronous operations back to back, where each subsequent operation starts when the previous operation succeeds, with the result from the previous step. We accomplish this by creating a promise chain.
So, changes that you need in you given code is
export function getUsers() {
return AsyncStorage.getItem('BASE_URL').then(url => {
return fetch(url+'/users').then(response => response.json());
});
}
You can use the getUsers()
without worrying about the BASE_URL
. This also ensures the separation of concern.
Hope this will help!
add a comment |
up vote
1
down vote
accepted
You can use promise chaining, where the result of AsyncStore#getItem
will be used as input to fetch
call. The linked document explain the situation that you are facing quite nicely
A common need is to execute two or more asynchronous operations back to back, where each subsequent operation starts when the previous operation succeeds, with the result from the previous step. We accomplish this by creating a promise chain.
So, changes that you need in you given code is
export function getUsers() {
return AsyncStorage.getItem('BASE_URL').then(url => {
return fetch(url+'/users').then(response => response.json());
});
}
You can use the getUsers()
without worrying about the BASE_URL
. This also ensures the separation of concern.
Hope this will help!
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can use promise chaining, where the result of AsyncStore#getItem
will be used as input to fetch
call. The linked document explain the situation that you are facing quite nicely
A common need is to execute two or more asynchronous operations back to back, where each subsequent operation starts when the previous operation succeeds, with the result from the previous step. We accomplish this by creating a promise chain.
So, changes that you need in you given code is
export function getUsers() {
return AsyncStorage.getItem('BASE_URL').then(url => {
return fetch(url+'/users').then(response => response.json());
});
}
You can use the getUsers()
without worrying about the BASE_URL
. This also ensures the separation of concern.
Hope this will help!
You can use promise chaining, where the result of AsyncStore#getItem
will be used as input to fetch
call. The linked document explain the situation that you are facing quite nicely
A common need is to execute two or more asynchronous operations back to back, where each subsequent operation starts when the previous operation succeeds, with the result from the previous step. We accomplish this by creating a promise chain.
So, changes that you need in you given code is
export function getUsers() {
return AsyncStorage.getItem('BASE_URL').then(url => {
return fetch(url+'/users').then(response => response.json());
});
}
You can use the getUsers()
without worrying about the BASE_URL
. This also ensures the separation of concern.
Hope this will help!
answered 2 days ago


Prasun
2,2542813
2,2542813
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53183816%2fvalue-from-async-function-in-url-inside-javascript-fetch%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
RmV,IxZcvJ5dd2fl0OPRSXAPvm16nnkqJA7bXftgOxaA1jKHBj3p73,f,KMmV A1
Why cant you pass the BASE_URL as parameter to the getusers function and call it inside .then of getItem?
– Shyam Babu
2 days ago
Ah. Nice idea. Thanks.
– Jithesh Kt
2 days ago